PROJECT: HMS+
This portfolio serves to document my contributions and learning while building HMS+ |
Overview
HMS+ is a hotel management system made for hotels to manage their customers and their relevant transactions. The hotel staff interacts with it using a Command Line Interface (CLI), and it has a Graphical User Interface (GUI) created with JavaFX. It is written in Java and has around 15,000 lines of code.
Summary of contributions
This section summarizes my contributions to the application and explains their importance. |
-
Major enhancement: I added the complete backend for the booking and reservation from commands up to storage. This also includes making sure each component is customizable which involved refactoring service and room types from
ENUM
to classes.-
What it does: It allows the hotel staff to add service-bookings, reservations, service types and room types to the hotel.
-
Justification: This is the backbone of the application as these are the main amenities of any hotel. Most other functionality such as bill and statistics can work because of these models.
-
Highlights: This enhancement required in-depth analysis of the codebase as it involved extending a single-model dependent system to a multi-model based system. I had to make sure the design implementation preserved the extensibility of the system as well as allowed the different components to interact with each other.
-
Credits: I mainly used the concepts from the existing codebase and those that were taught in CS2103T to implement my features.
-
Related Files:
-
-
Minor enhancements:
-
Other contributions:
-
Project management:
-
Created the Github Project Board and facilitated a issue-based workflow.
-
-
Enhancements to existing features:
-
Set the design structure for the implementation of future models.
-
Created utility classes to handle timings and date intervals with relevant tests.
-
-
Documentation:
-
Added command formats and descriptions to the user guide.
-
Added color-coding to the user guide.
-
Reformatted all the commands to match an intuitive convention in guides and codebase.
-
Added sections to developer guide. Example- I added a section to teach oncoming developers how to add new models to the codebase.
-
-
Tools:
-
Set Up Travis, Codacy and Coveralls
-
Set the minimum requirements for code to be merged to master.
-
-
Contributions to the User Guide
This section displays the main sections of the User Guide added or edited by me. They showcase my ability to write documentation which can be understood by the targeted end-users. |
Room Reservation commands
Reserving a room : add-reservation
, ar
Effect: Adds a reservation for a room associated with certain customers.
Format: {add-reservation/ar} r/ ROOM_TYPE d/ START_DATE-END_DATE $/ PAYER_INDEX [ c/ MORE_CUSTOMER_INDICIES… ] [ com/ COMMENTS ]
|
Examples:
Assume current date is 10 May, 2019.
-
list-customers
, thenar r/SINGLE ROOM d/20/5/2019-25/5/2019 $/5
Adds a reservation of Single Room in the name of the 5th customer from 20 May 2019 to 25 May 2019.
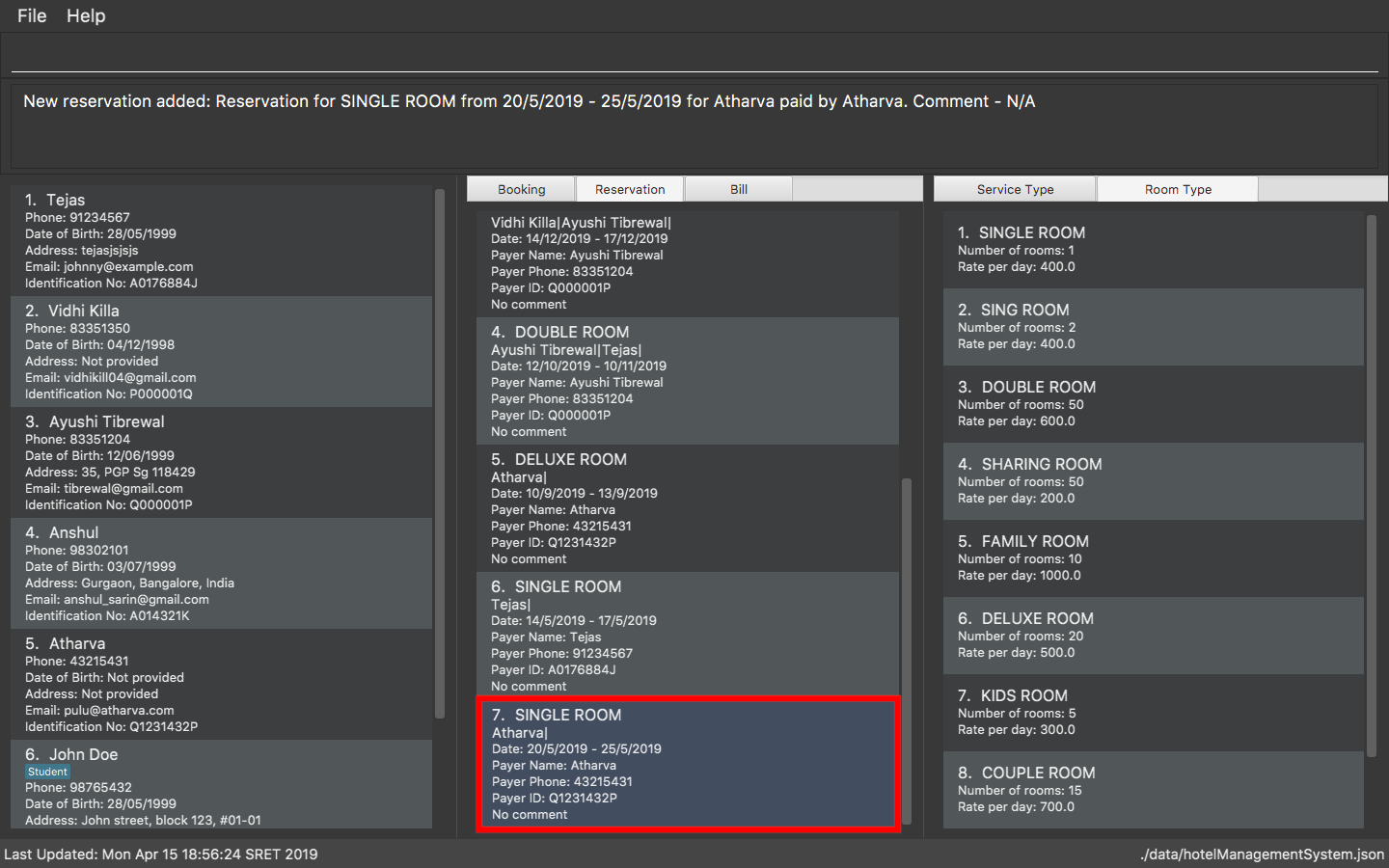
above
command-
list-customers
, thenar r/SHARING ROOM d/23/5/2019-25/5/2019 $/4 c/2 c/3
Adds a reservation of Sharing Room in the name of the 4th customer along with the 2nd and 3rd customers from 23 May 2019 to 25 May 2019.
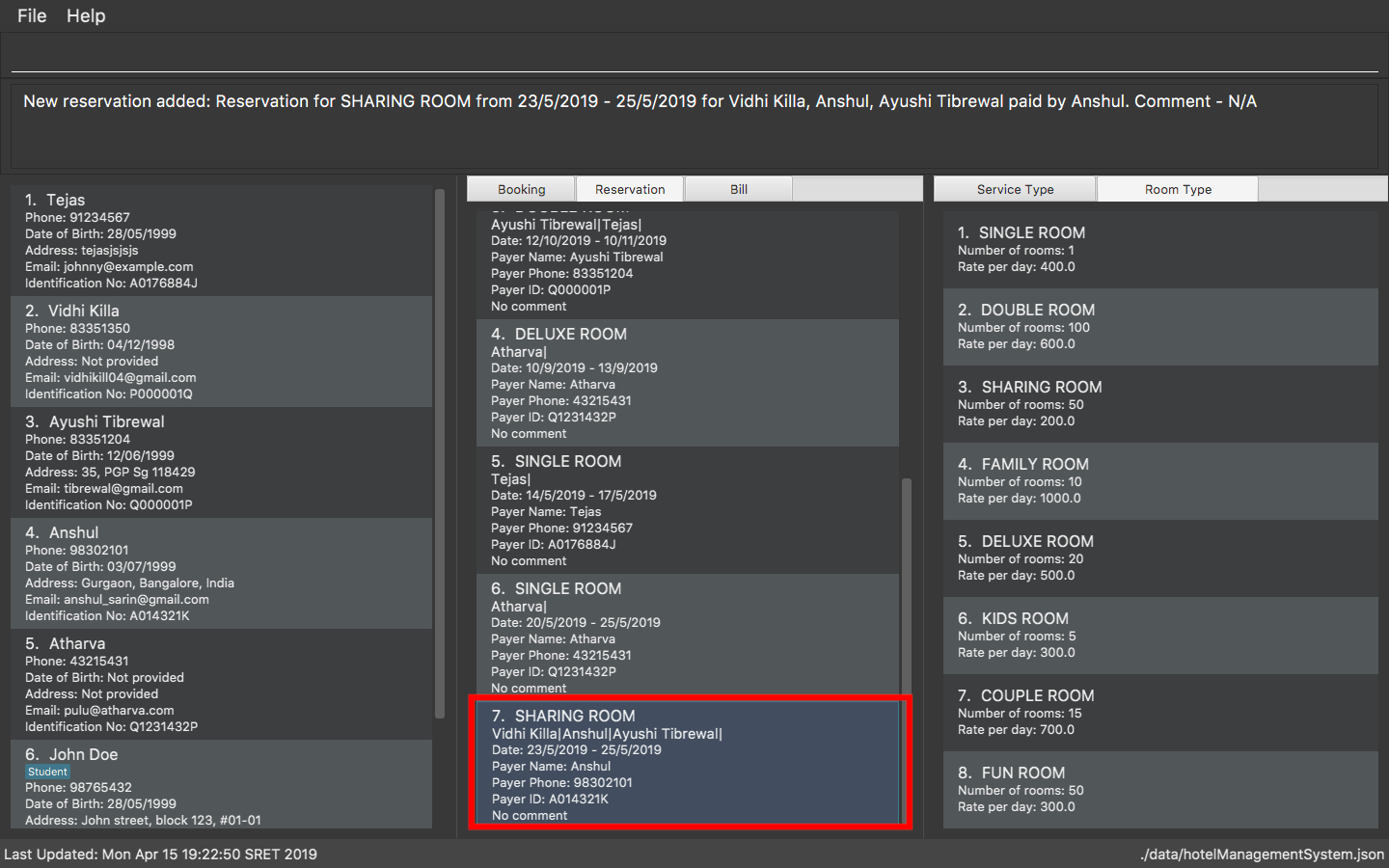
above
commandListing all reservations : list-reservations
, lr
Effect: Displays a reservation list, which lists all the reservations.
Format: {list-reservations/lr}
Examples:
-
lr
Lists all reservations.
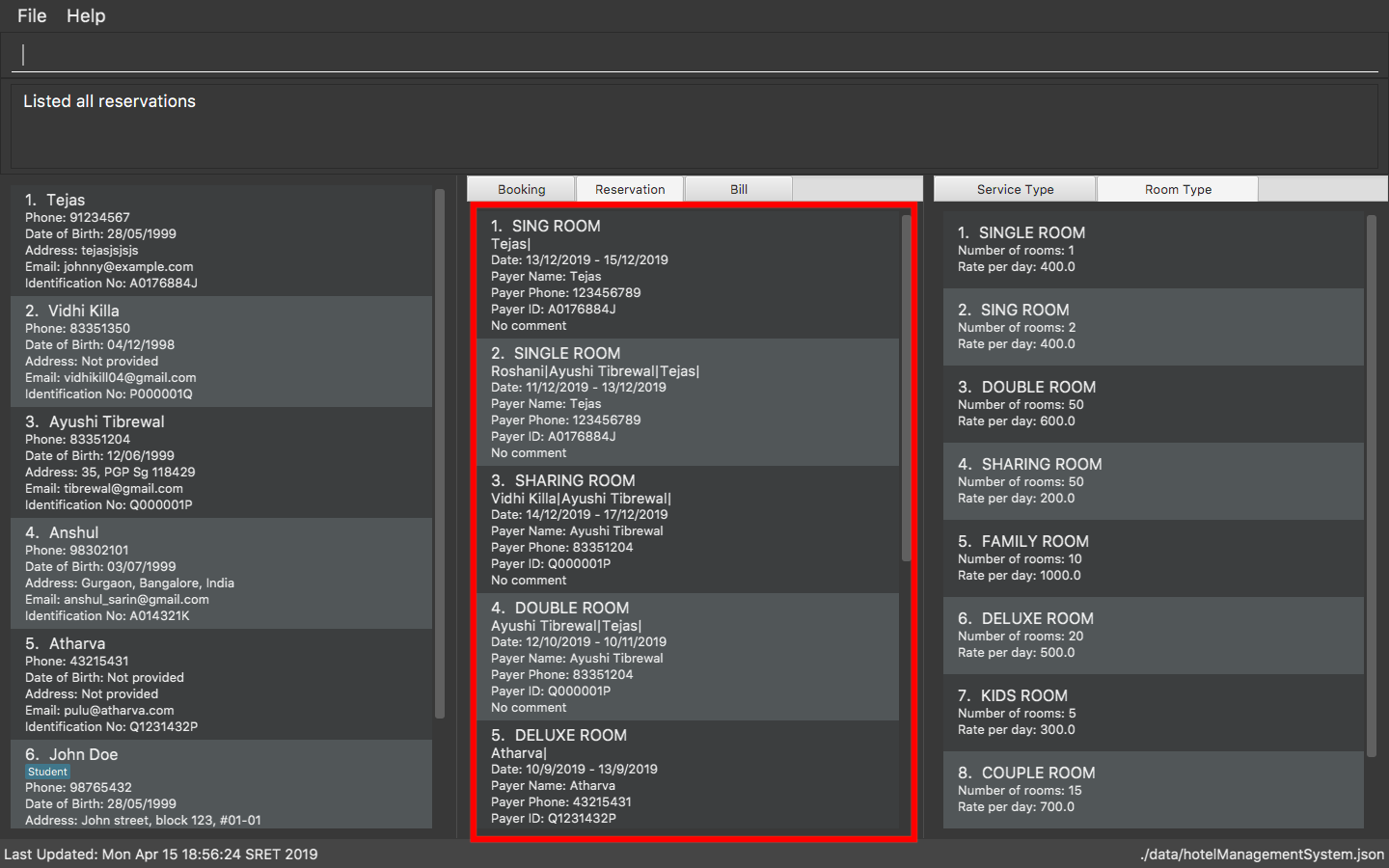
lr
commandEditing a room reservation : edit-reservation
, er
Effect: Edits the fields of an existing reservation in the reservation database.
Format: {edit-reservation/er} INDEX [ r/ ROOM_TYPE ] [ d/ START_DATE-END_DATE] ] [ $/ PAYER_INDEX ] [ c/ MORE_CUSTOMER_INDICES ] [ com/ COMMENTS ]
|
You can remove all the reservation’s comments by typing com/ without specifying any tags after it.
|
Examples:
-
lr
, thener 1 r/DOUBLE ROOM
Edits the room type of the 1st reservation to be DOUBLE ROOM.
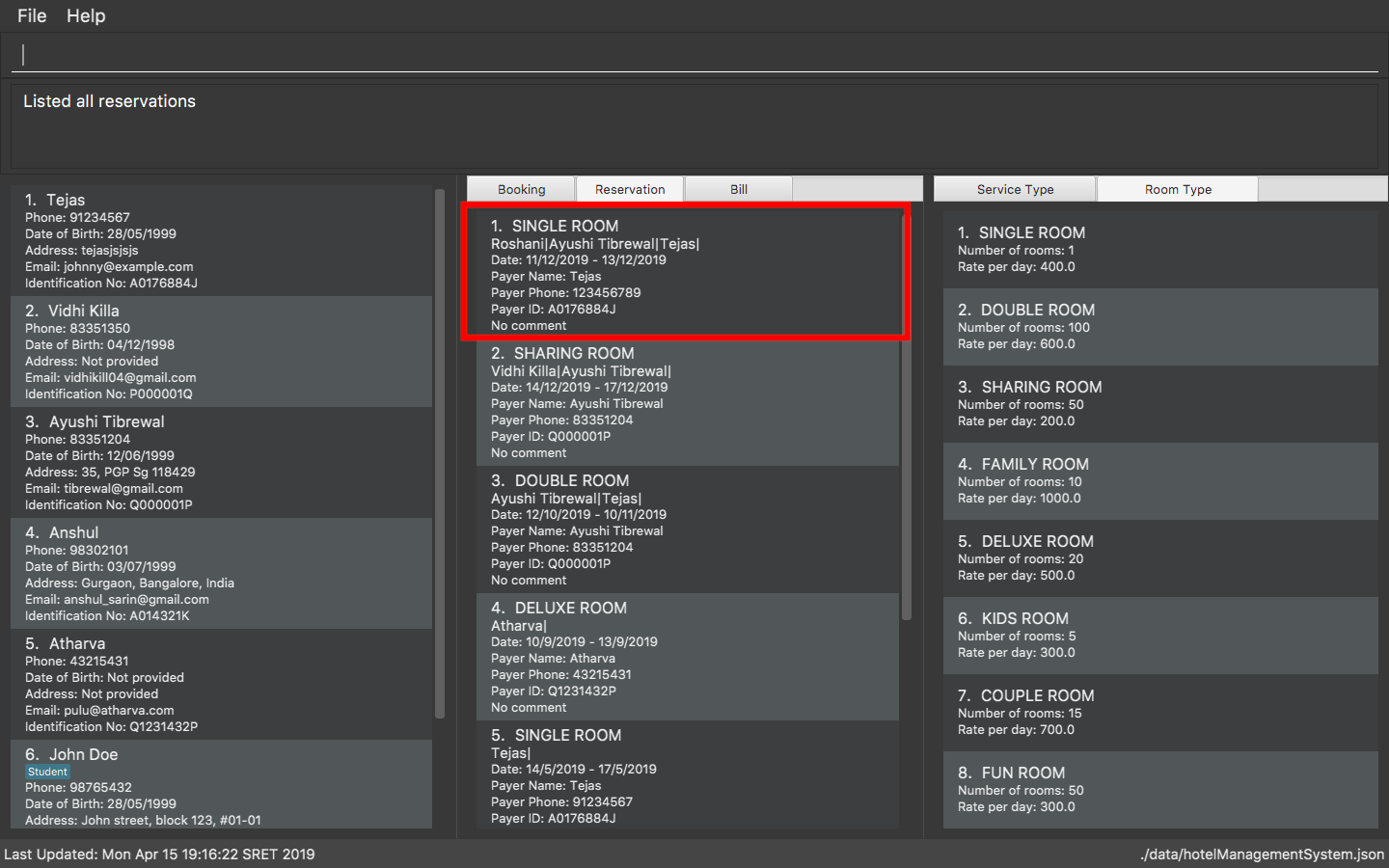
er 1 r/DOUBLE ROOM
command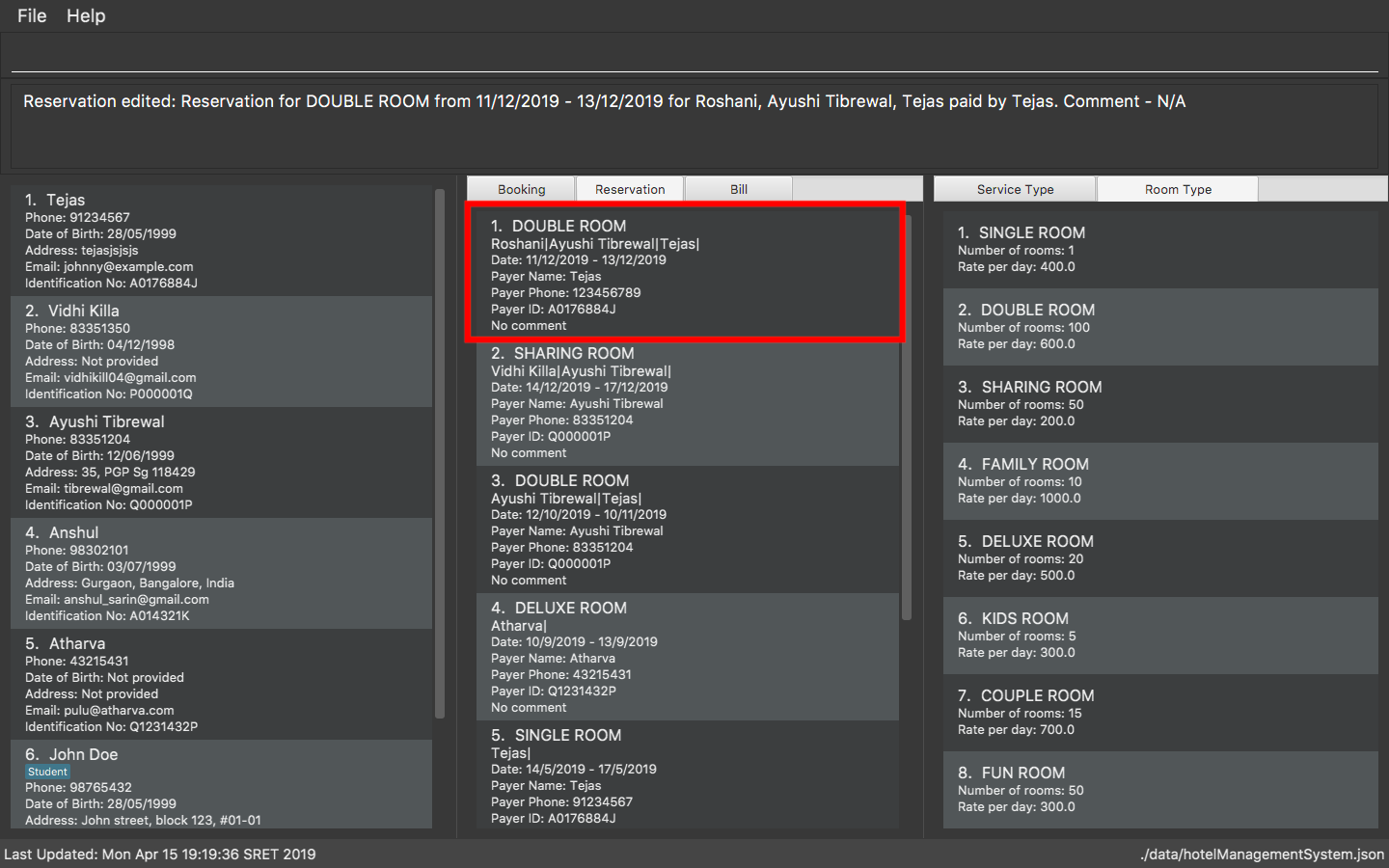
er 1 r/DOUBLE ROOM
command-
lr
, thener 2 d/14/02/2020-14/03/2020 com/
Edits the date of the 2nd reservation to be from 14 Feb 2020 to 14 Mar 2020 and clears all existing comments related to it.
Deleting reservations: delete-reservation
, dr
Effect: Deletes a reservation from the reservation database.
Format: {delete-reservation/dr} INDEX
Examples:
-
lr
, thendr 2
Deletes the 2nd entry of the reservation database.
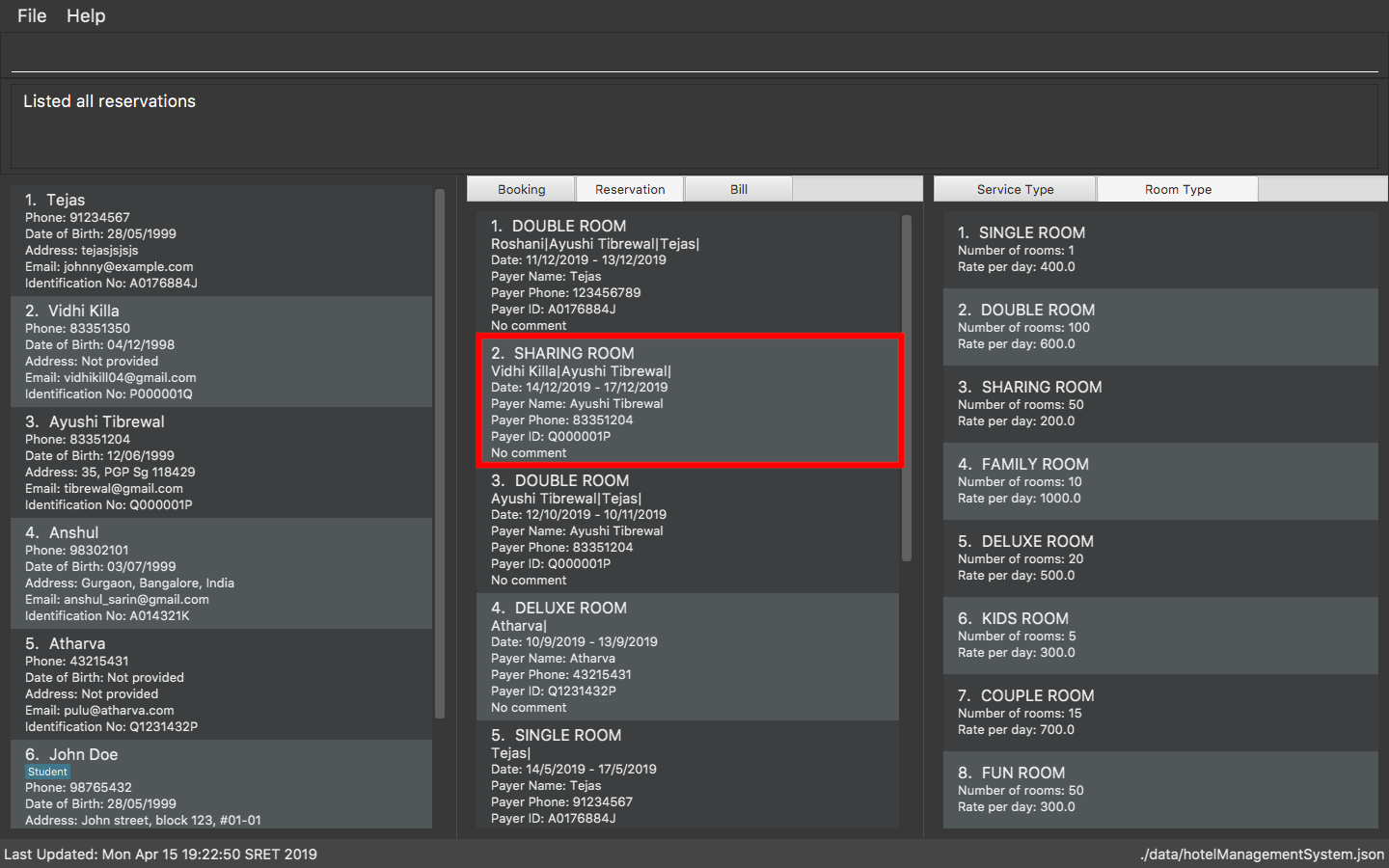
dr 2
command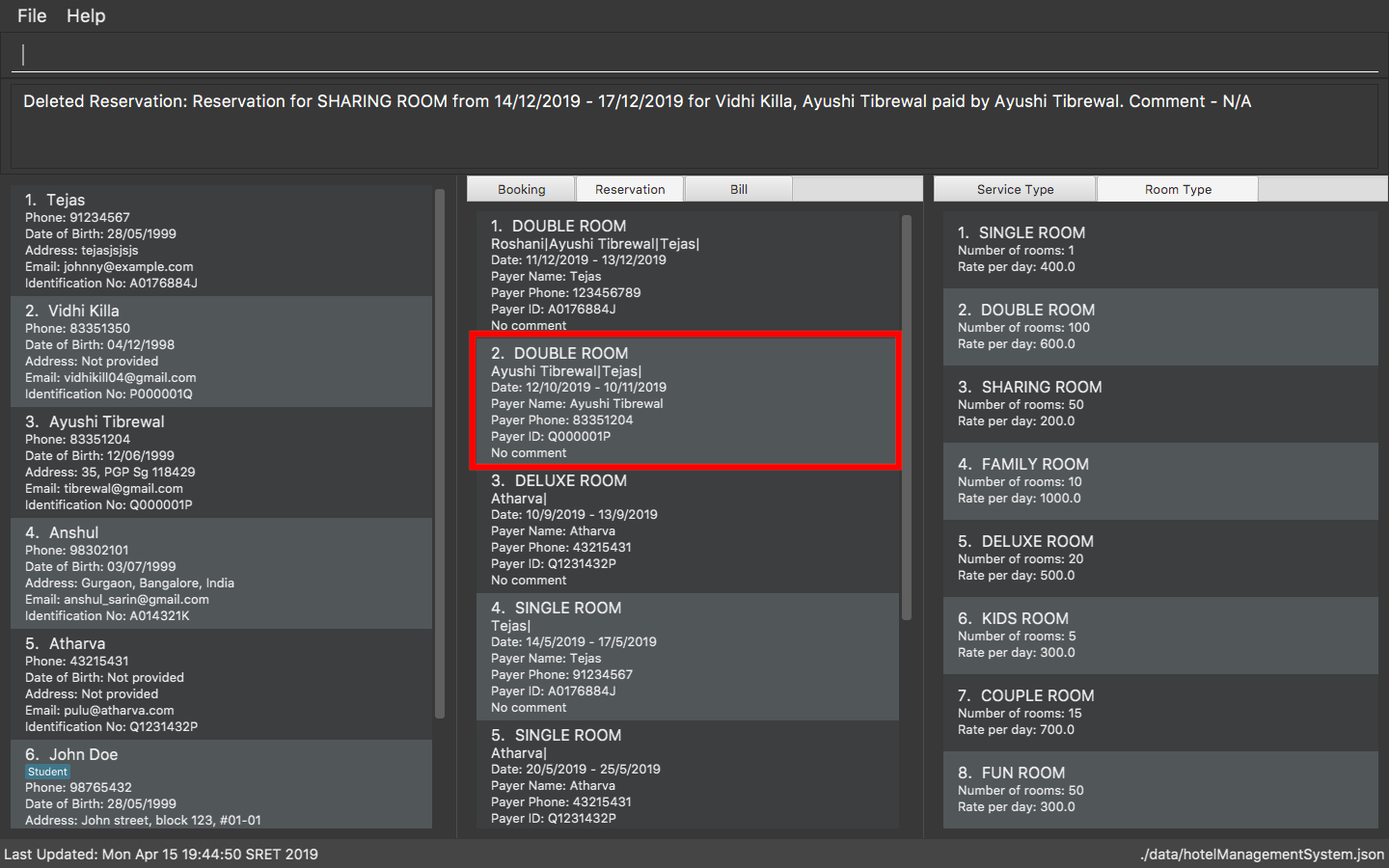
dr 2
commandClearing all reservations: clear-reservations
, cr
Effect: Removes all room reservations from the database.
Format: {clear-reservations/cr}
Examples:
-
cr
Clears all reservations from the database.
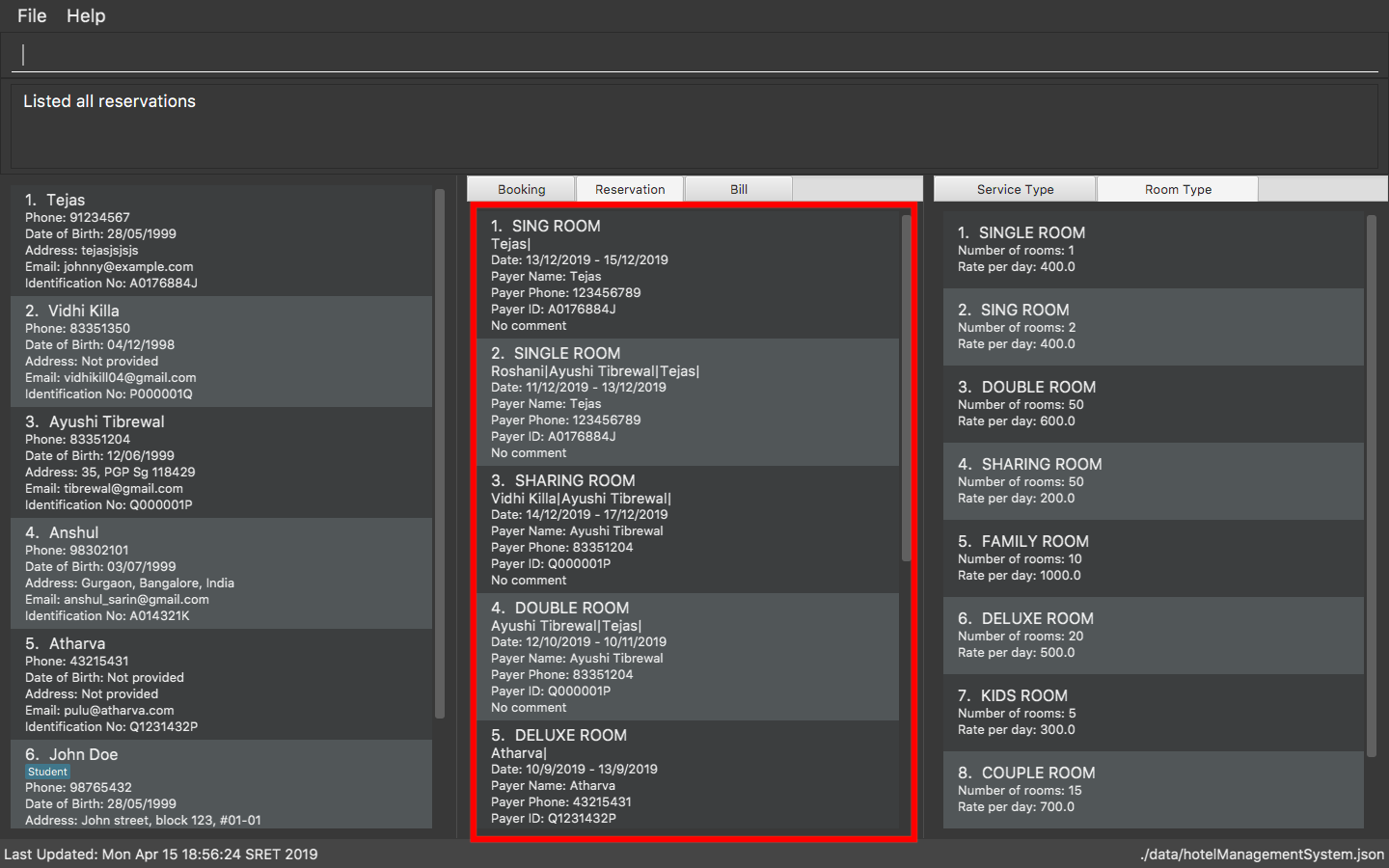
cr
command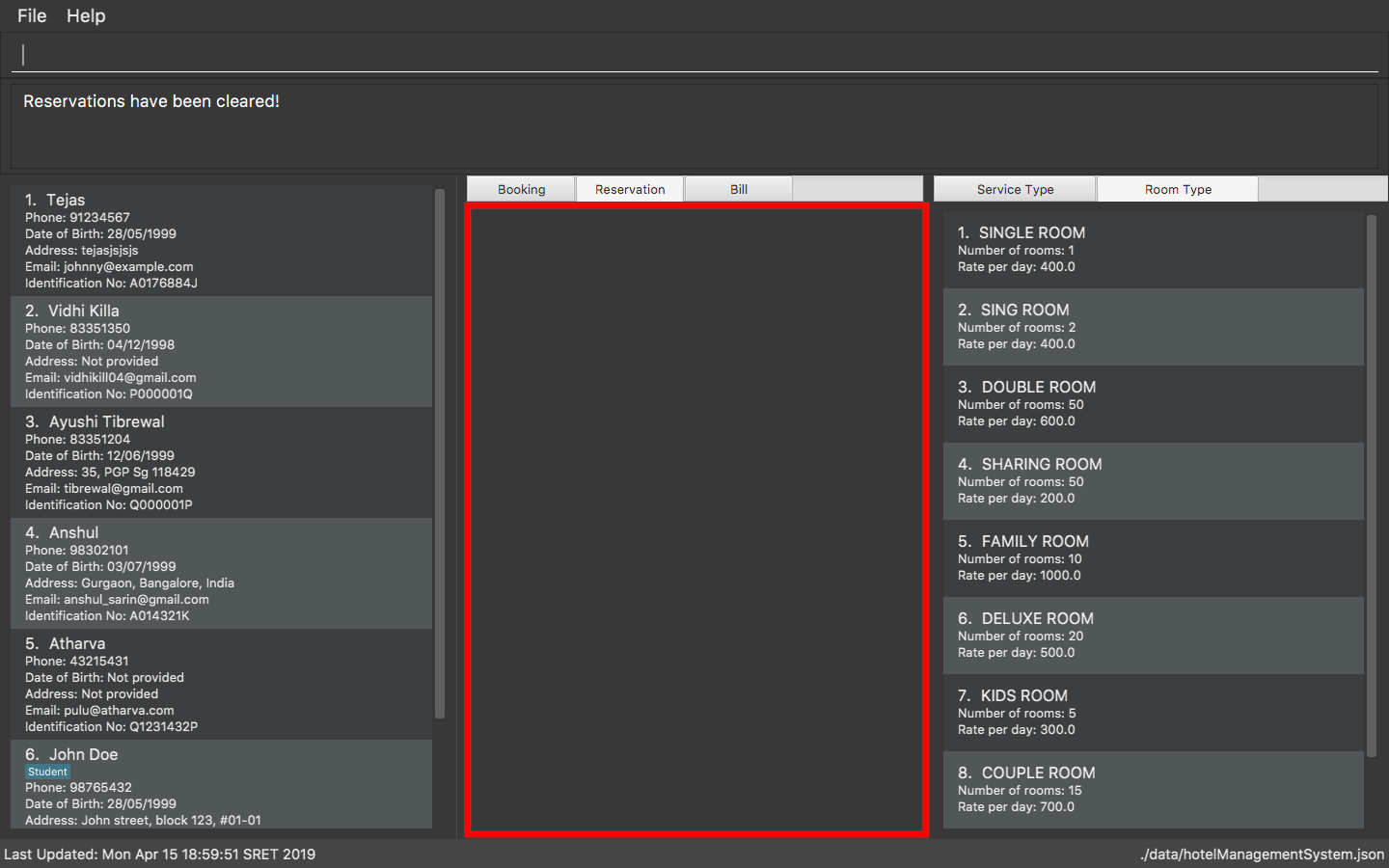
cr
commandFind a reservation: find-reservation
, fr
Effect: Displays a reservation list based on the filters given by the user.
Format: {find-reservation/fr} [ id/ IDENTIFICATION_NO ] [ r/ ROOM_TYPE ] [ d/ START_DATE - END_DATE ]
|
|
Example:
-
fr id/Q000001P
Returns all reservations for customer with identification number, Q000001P.
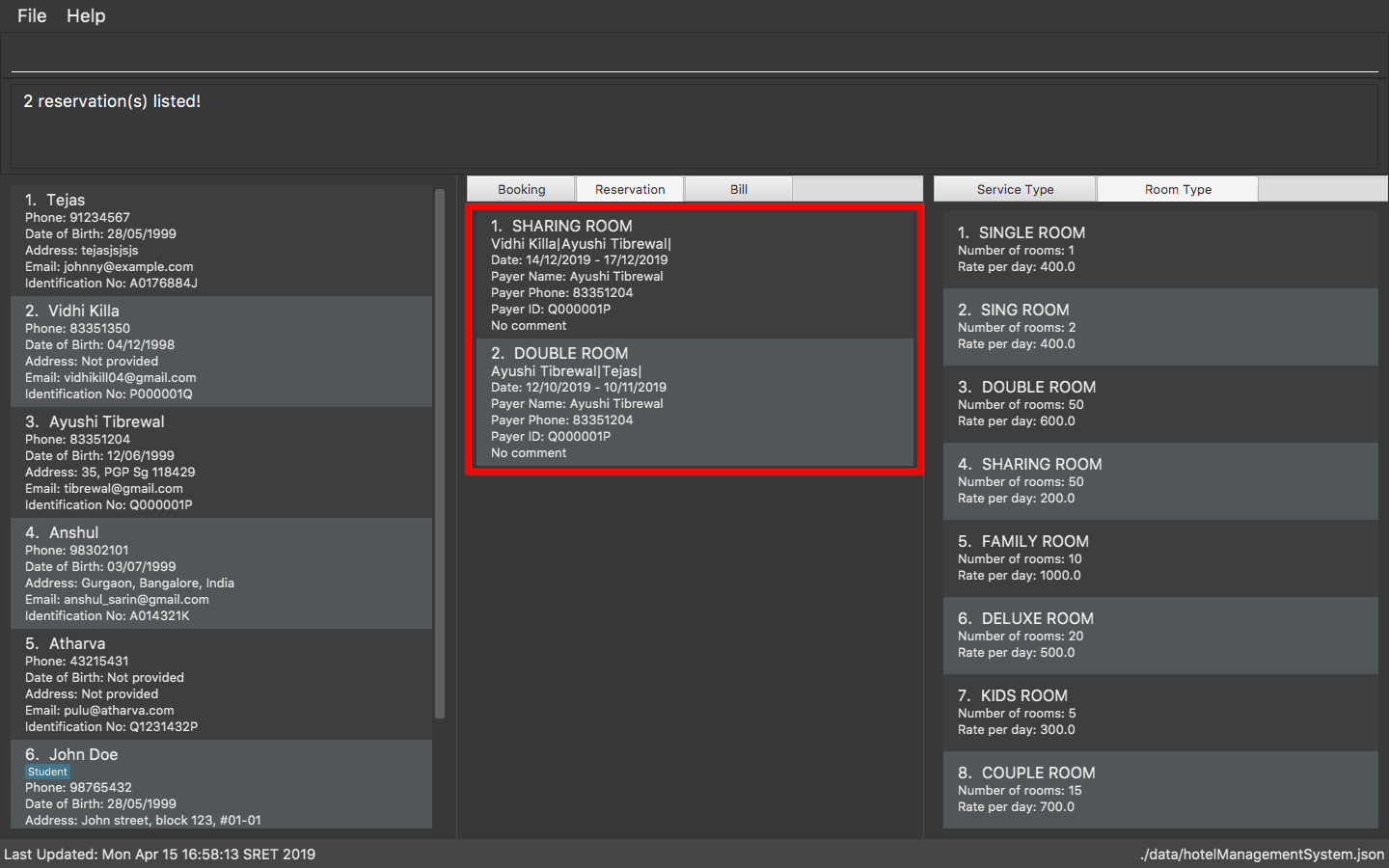
fr id/Q000001P
command-
fr id/Q000001P r/SHARING ROOM
Returns all sharing room reservations for customer with identification number, Q000001P.
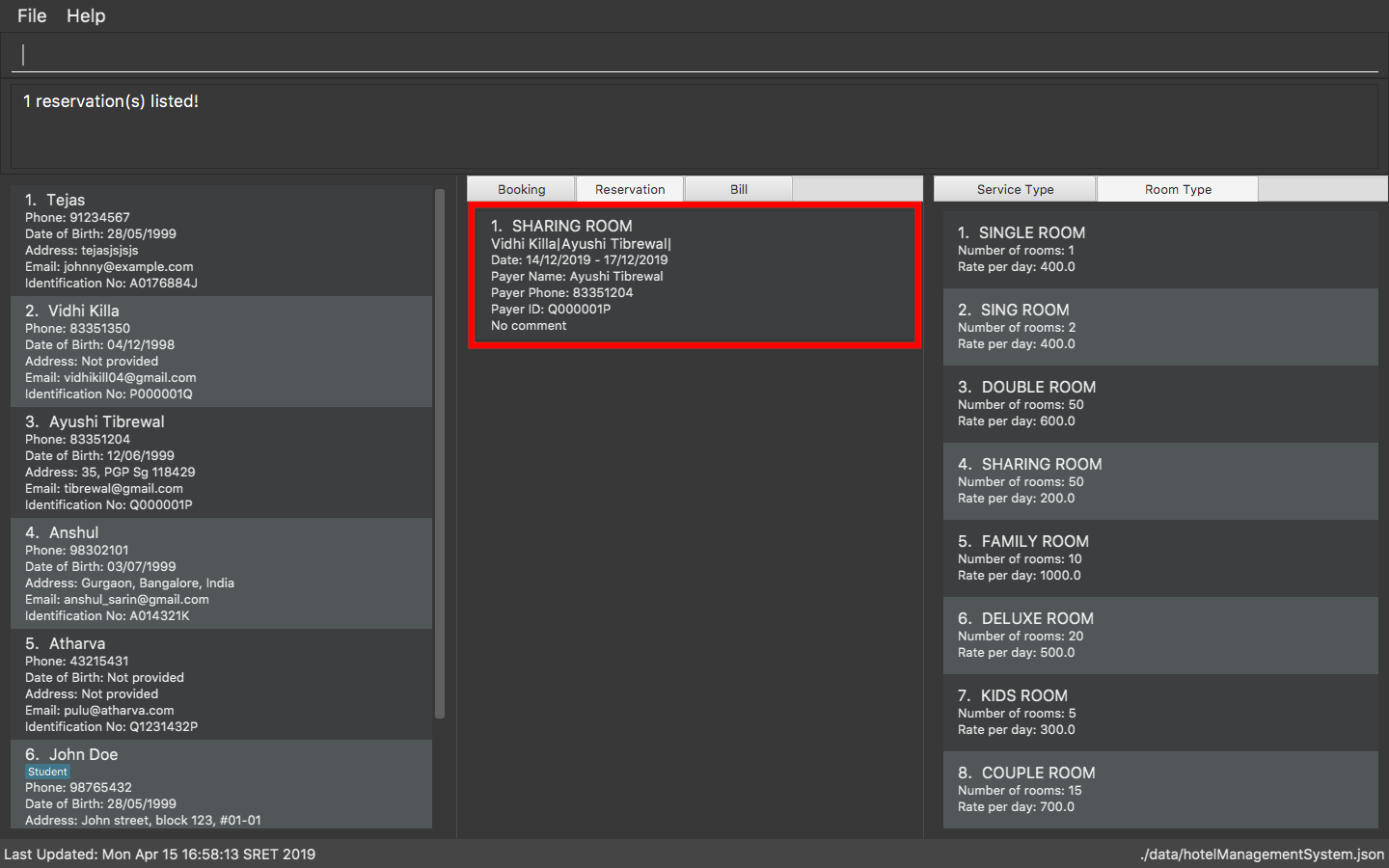
fr id/Q000001P r/SHARING ROOM
commandService Booking commands
Booking a service: add-booking
,ab
Effect: Adds a service associated with certain customers.
Format: {add-booking/ab} s/ SERVICE_NAME :/ START_TIME-END_TIME $/ PAYER_INDEX [ c/ MORE_CUSTOMER_INDICES ] [ com/ COMMENTS ]
|
Examples:
-
lc
, thenadd-booking s/SWIMMING POOL :/12-14 $/2
Adds a booking for service SWIMMING POOL, for the 2nd customer from the complete customer list, from 12:00 to 14:00 if the service is available.
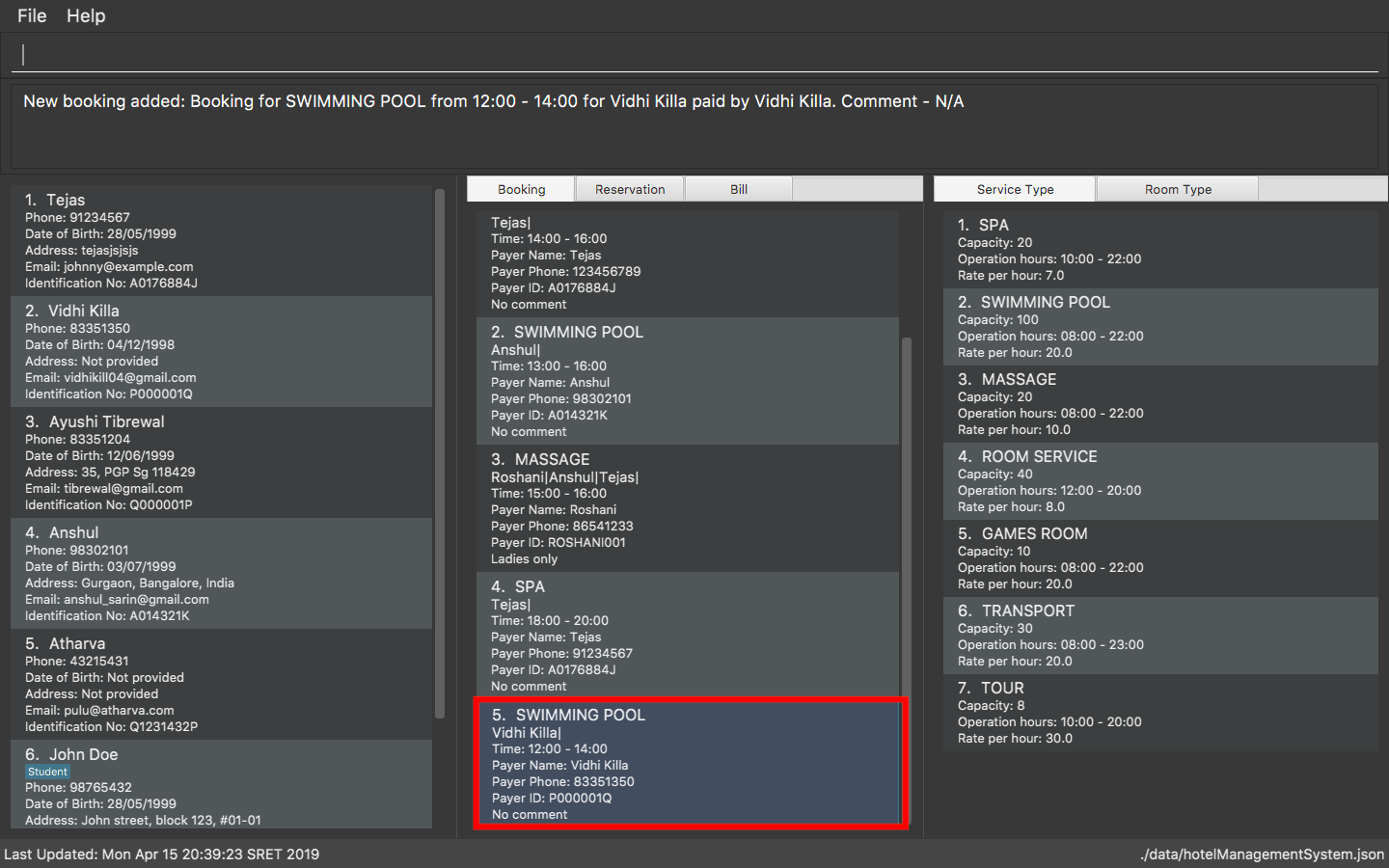
above
commandListing all booked services: list-bookings
,lb
Effect: Displays a booking list, which lists all the bookings made till now.
Format: {list-bookings/lb}
Example:
-
lb
Lists all bookings.
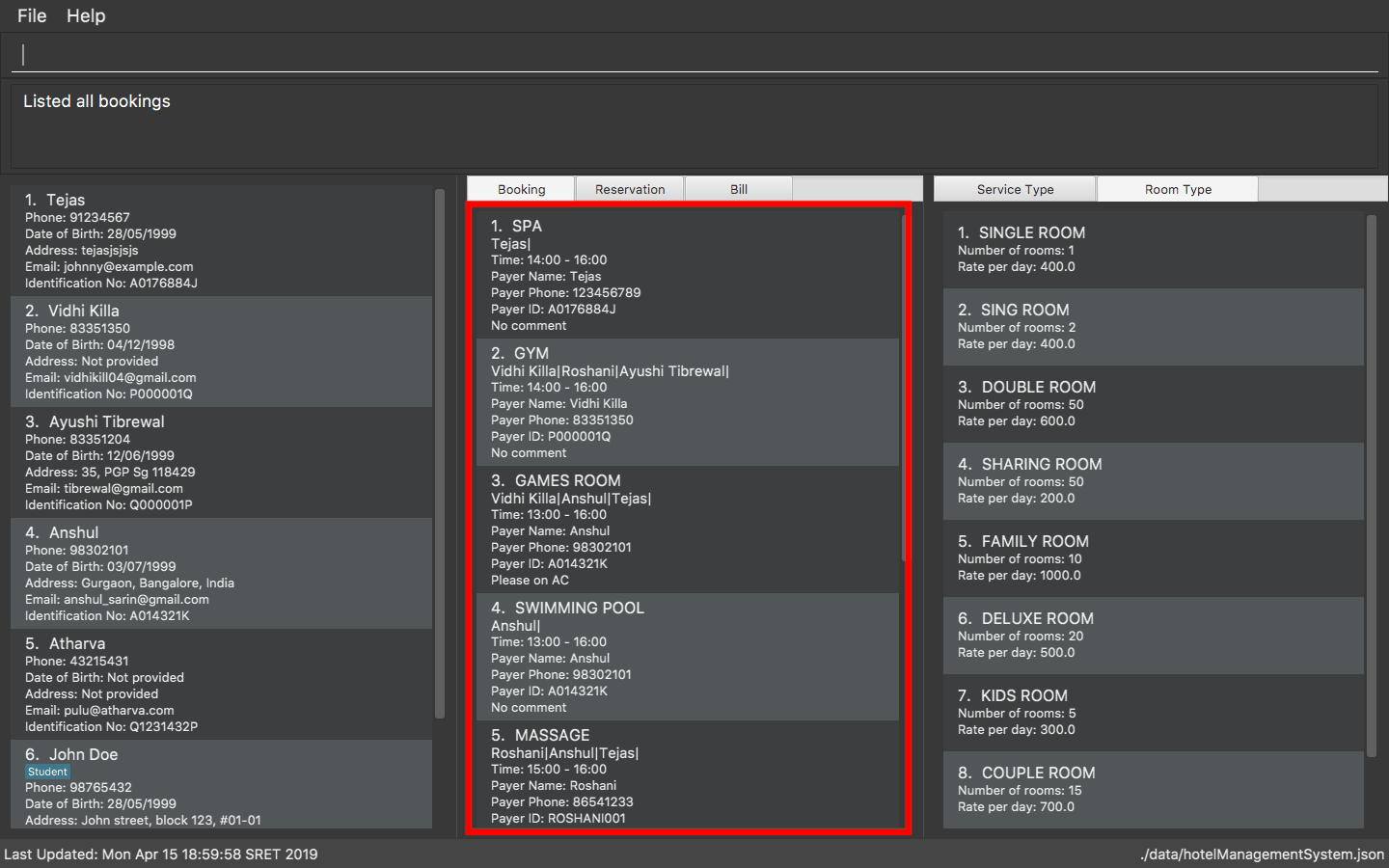
lb
commandEditing a booked service: edit-booking
, eb
Effect: Edits the fields of a booking in the database.
Format: {edit-booking/eb} INDEX [ s/ SERVICE_NAME ] [ :/ START_TIME-END_TIME ] [ p/ PAYER_INDEX ] [ c/ MORE_CUSTOMER_INDICES ] [ com/ COMMENTS ]
|
You can remove all the booking’s comments by typing com/ without specifying any tags after it.
|
Examples:
-
lb
, theneb 1 s/TRANSPORT
Edits the service type of the 1st booking to be GYM.
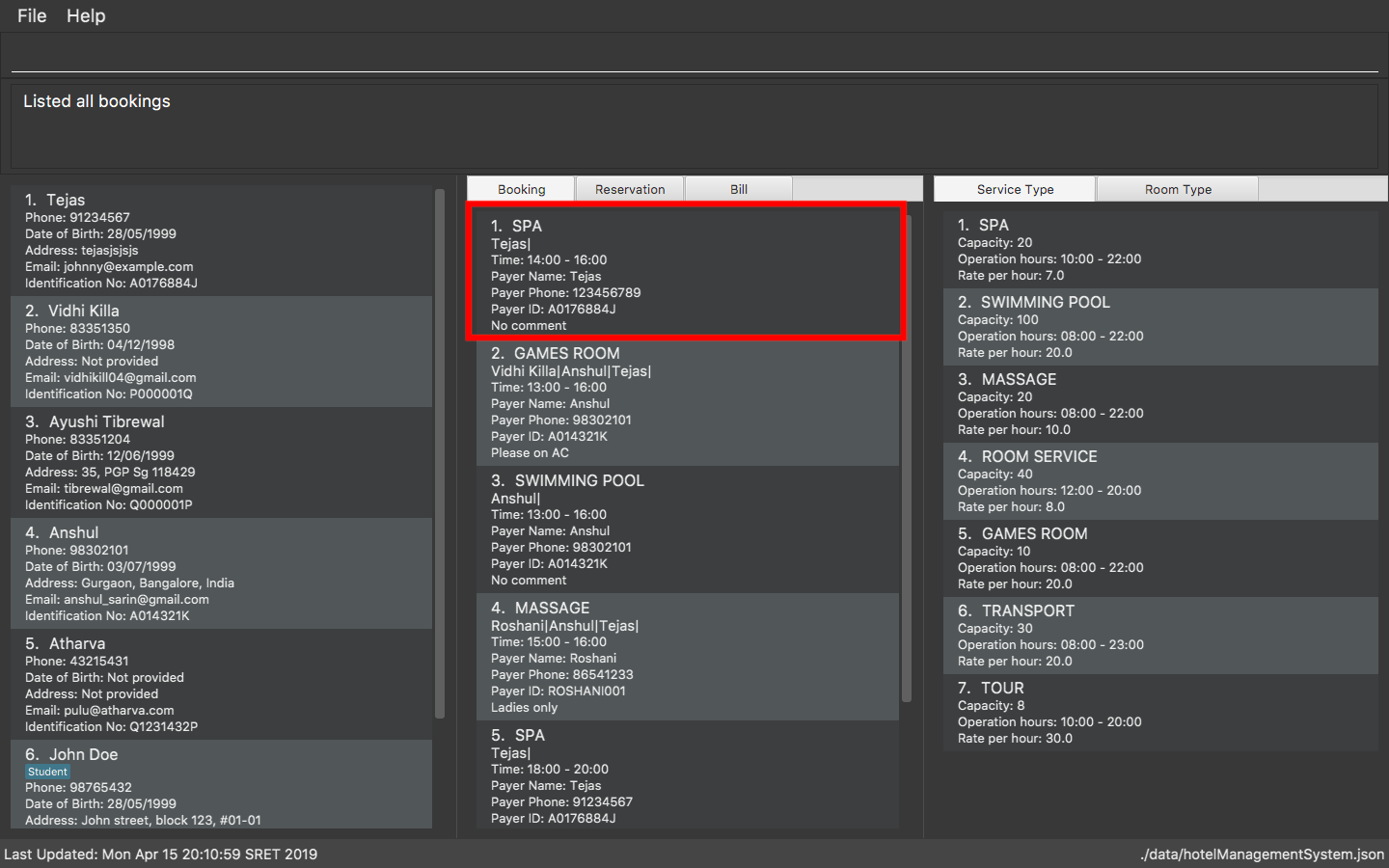
eb 1 s/TRANSPORT
command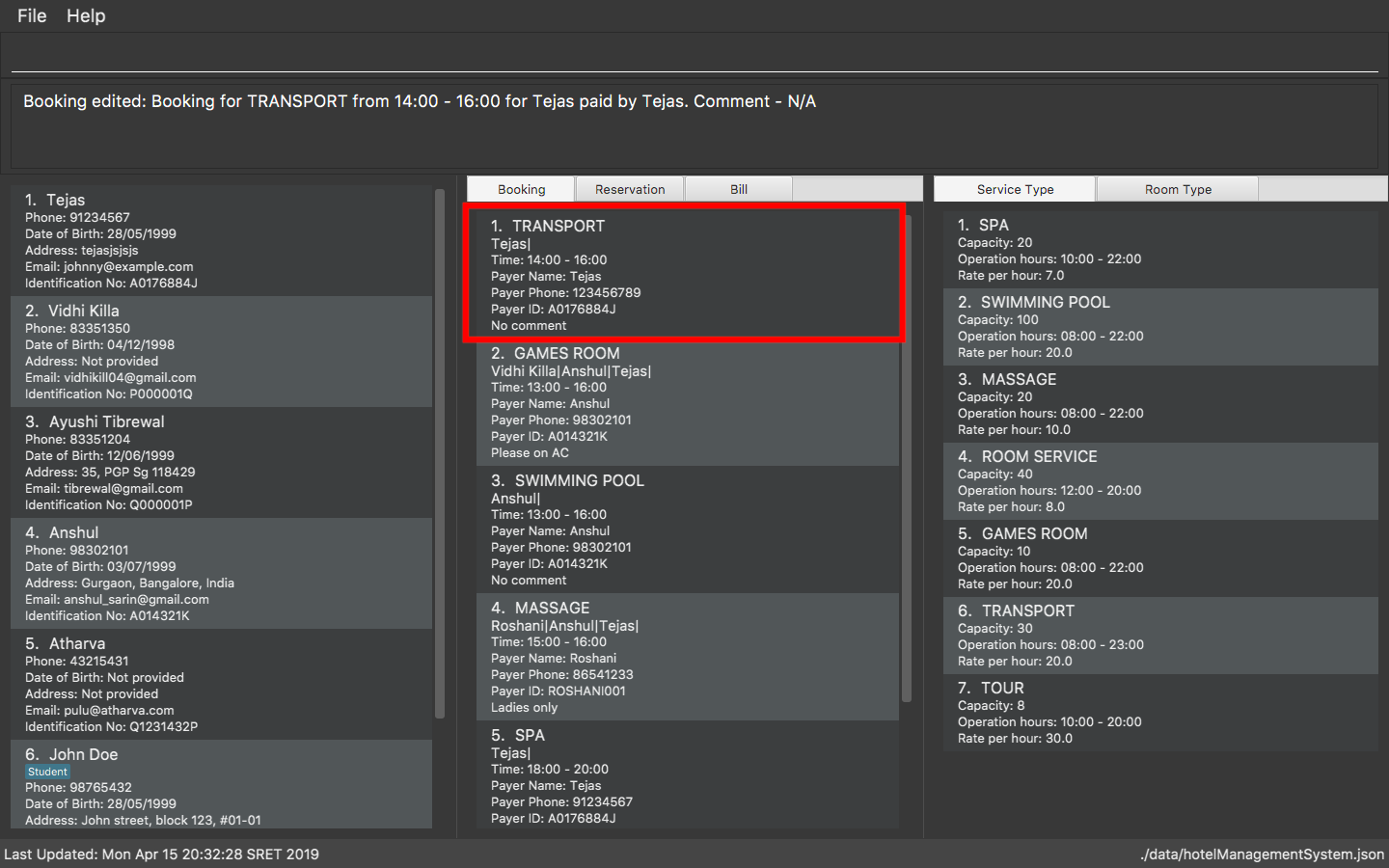
eb 1 s/TRANSPORT
command-
lb
, thenedit-booking 2 :/14-15 com/
Edits the timing of the 2nd booking to be 14:00 - 15:00 and clears all existing comments.
Deleting a booked service: delete-booking
, db
Effect: Deletes a booking from the database.
Format: {delete-booking/db} INDEX
|
Example:
lb
, then delete-booking 2
Deletes the 2nd booking of the booking database
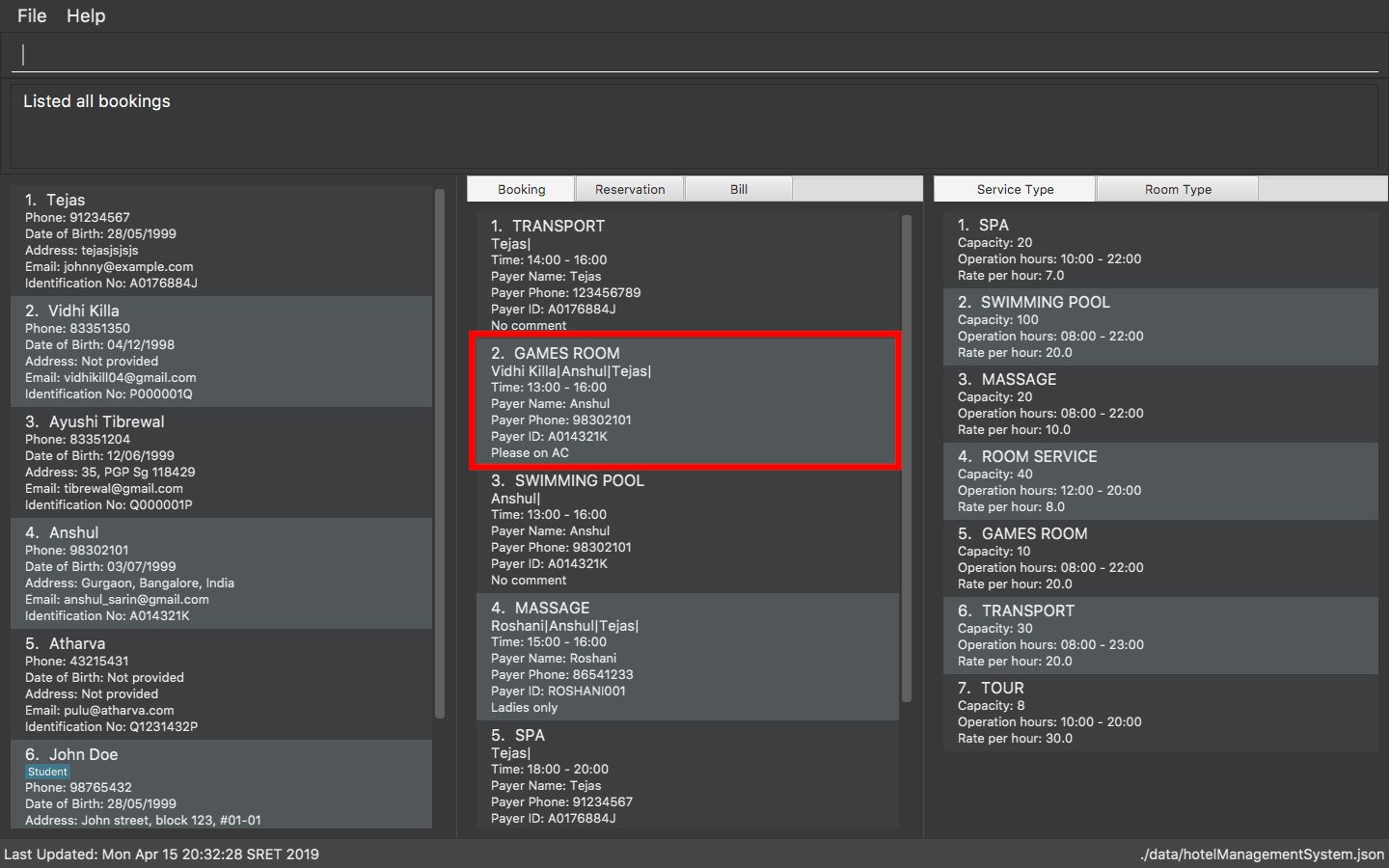
delete-booking 2
command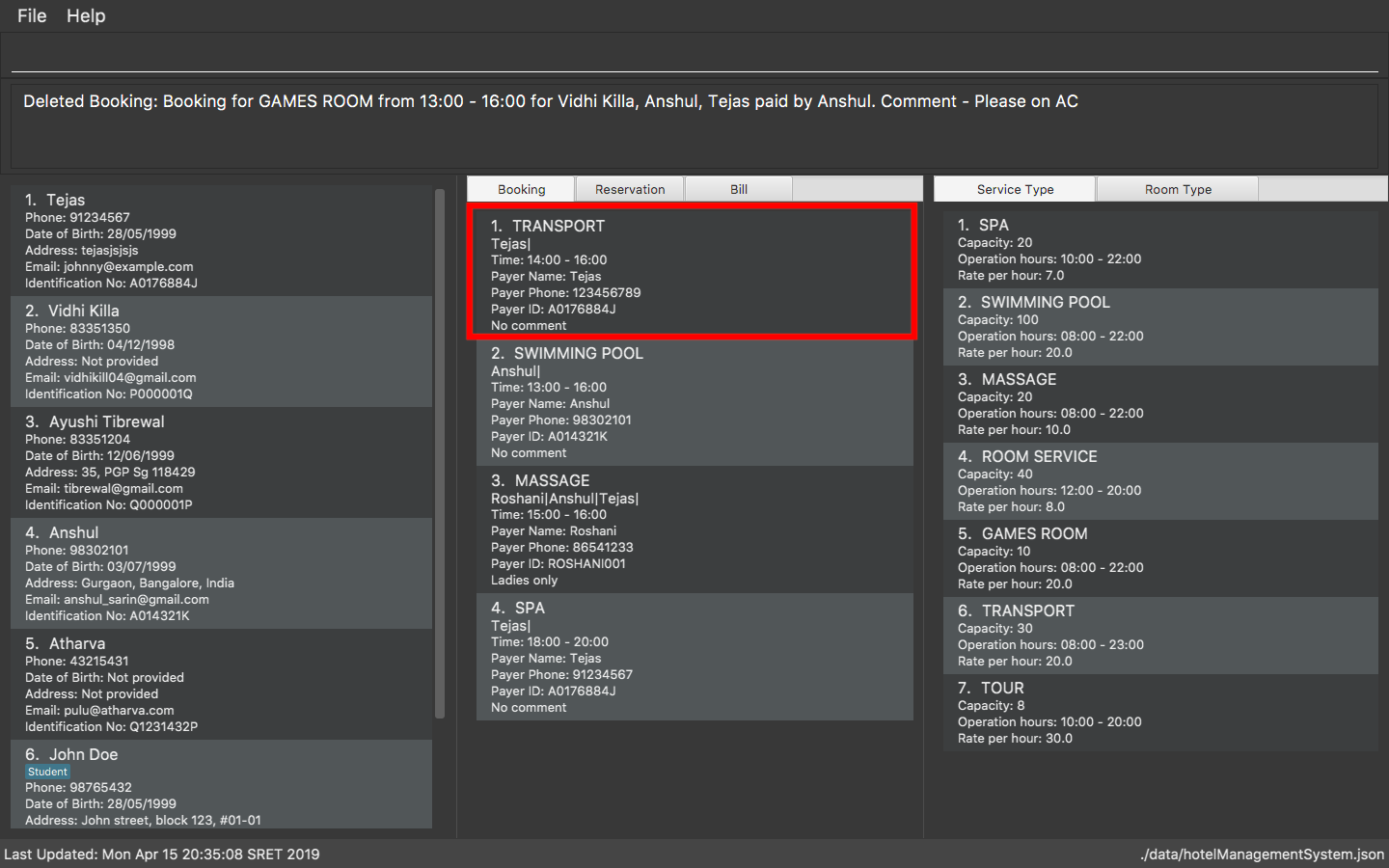
delete-booking 2
commandClearing all bookings: clear-bookings
, cb
Effect: Removes all service bookings from the database.
Format: {clear-bookings/cb}
Example:
-
cb
Clears all bookings from the database.
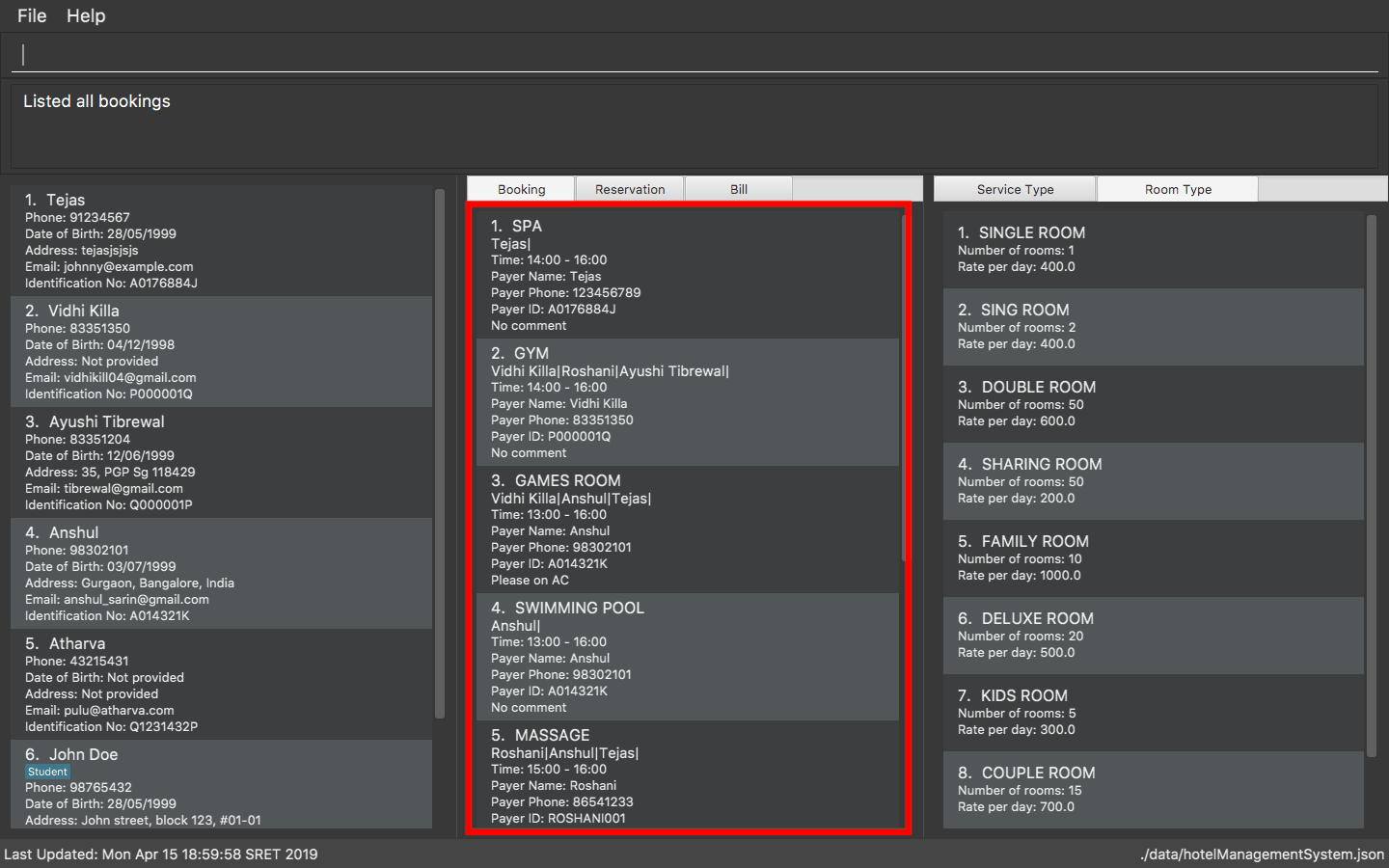
cb
command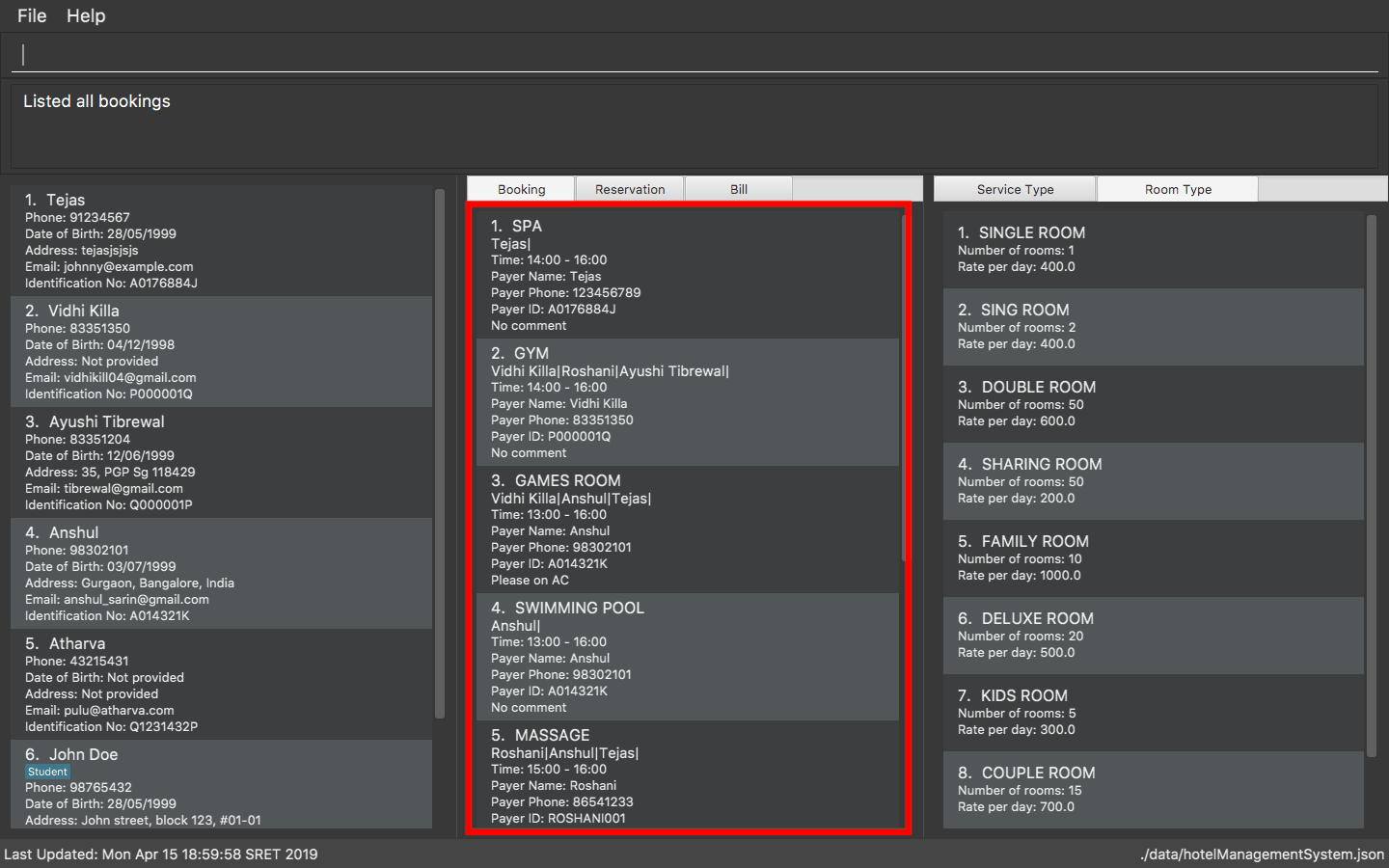
cb
commandFind a specific booking: find-booking
, fb
Effect: Displays a booking list based on the filters given by the user.
Format: {find-booking/fb} [ id/ IDENTIFICATION_NO ] [ s/ SERVICE_NAME ] [ :/ START_TIME-END_TIME ]
|
Example:
-
fb id/A0176884J
Returns all bookings for customer with identification number, A0176884J.
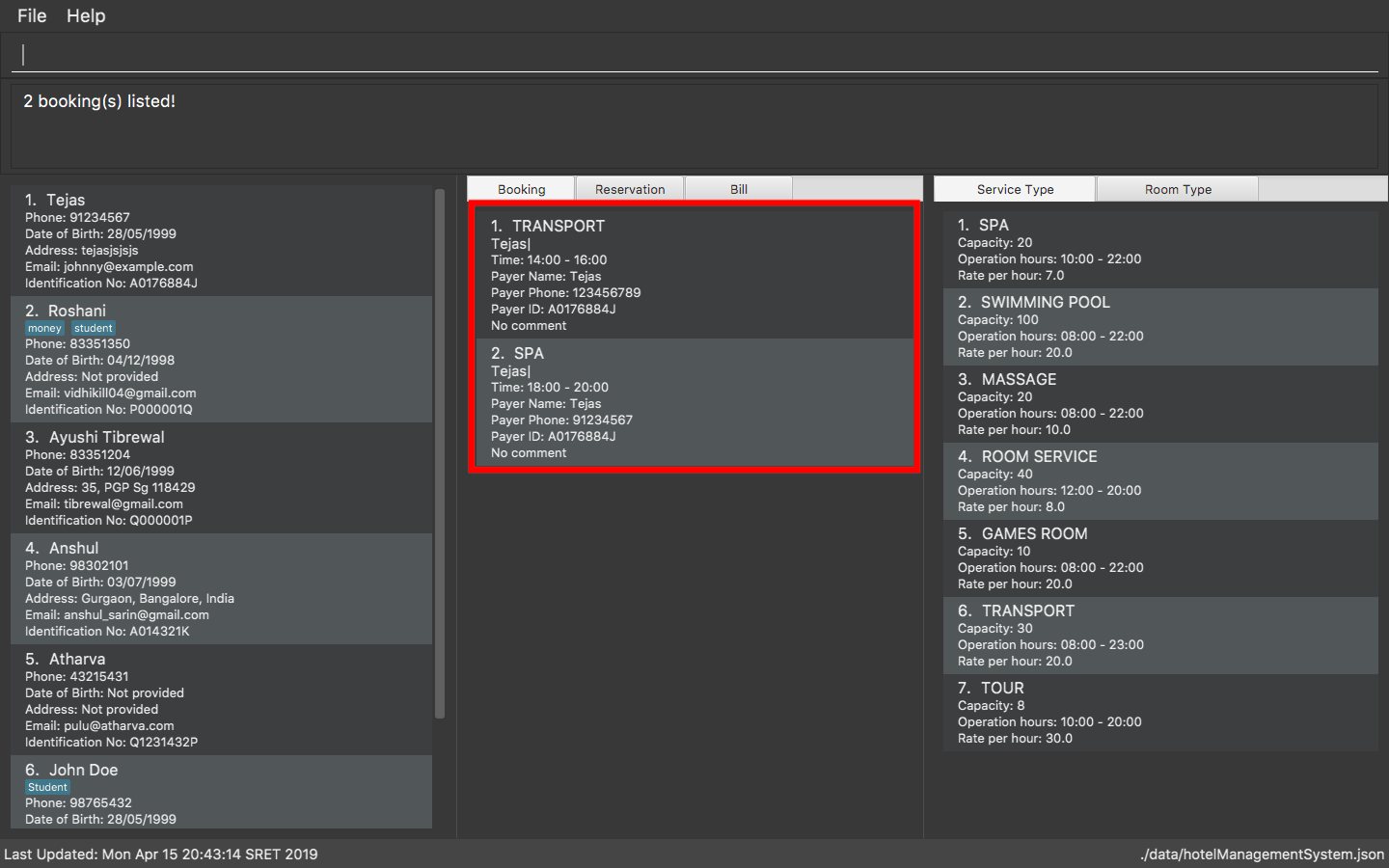
fb id/A0176884J
command-
fb id/A0176884J s/SPA
Returns all spa bookings for customer with identification number, A0176884j.
Contributions to the Developer Guide
Given below are the main sections I added to the developer guide so that oncoming developers can easily extend our codebase and add features quickly. |
How to include a new model
Current Implementation using service booking
In general, when adding any new model and its related commands, you need to work on 3 components compulsorily - Logic, Model and Storage. The UI component is modified based on how you want the user to be able to interact with our newly added model.
You will start by working on the model component. You must create a more specific model which
implements the existing Model
interface and create a manager for this model. This constitutes
the API for our new model how the Logic
component will execute the commands related to the model.
For Booking, there is a BookingModel
interface and a BookingManager
class which
keeps track of a booking list. An 'observable booking list' is bounded to the UI using
listeners so that the UI can be updated as the list changes. While the interface is a means of exposing the
API, the manager class links with the system and performs the actions. We also create the relevant class files
to represent the model we are implementing. For example, for representing our list of reservations,
we create relevant classes like ReservationList
, Reservation
, DateRange
, RoomType
, etc.\
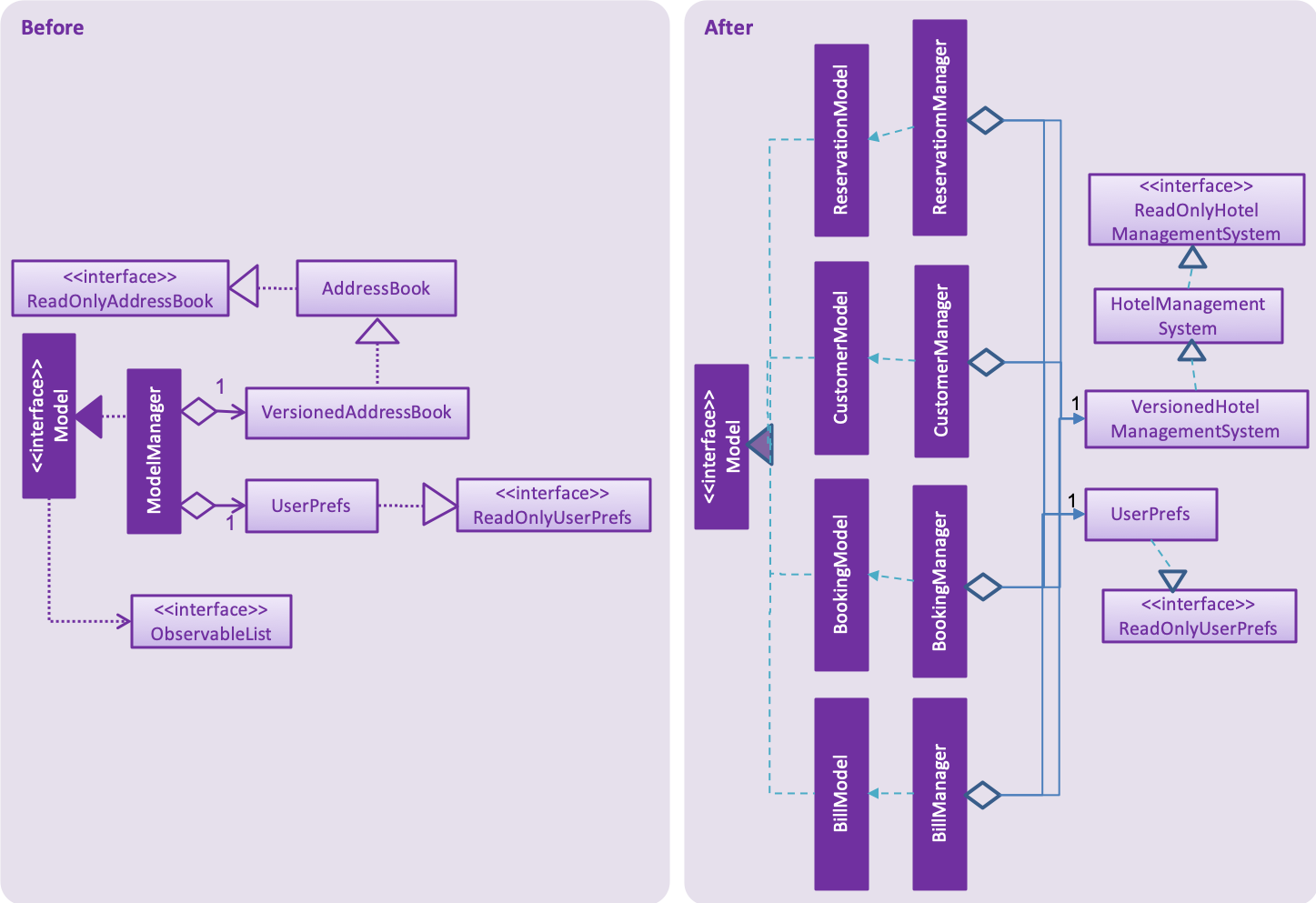
Then you need to add the command files and parser files so that the application can parse commands
related to the model and execute them. The commands have been separated for different models
by adding an interface in the middle. This interface makes sure the correct model is used to execute
those commands. For example, there is a CustomerCommand
and a BookingCommand
interface which
implements the Command
interface. Moreover the CustomerCommand
interface specifies that it uses
the CustomerModel
to execute itself. Then each command (eg. AddBookingCommand, EditBookingCommand, etc.)
implements the BookingCommand
interface. This makes sure that only the booking manager can be used to
execute these commands as well. You also need to add parser classes for each command you implement
(eg. AddBookingCommandParser). These classes are invoked in the HotelManagementSystemParser
to get back the CommandResult
which is used by the UI.
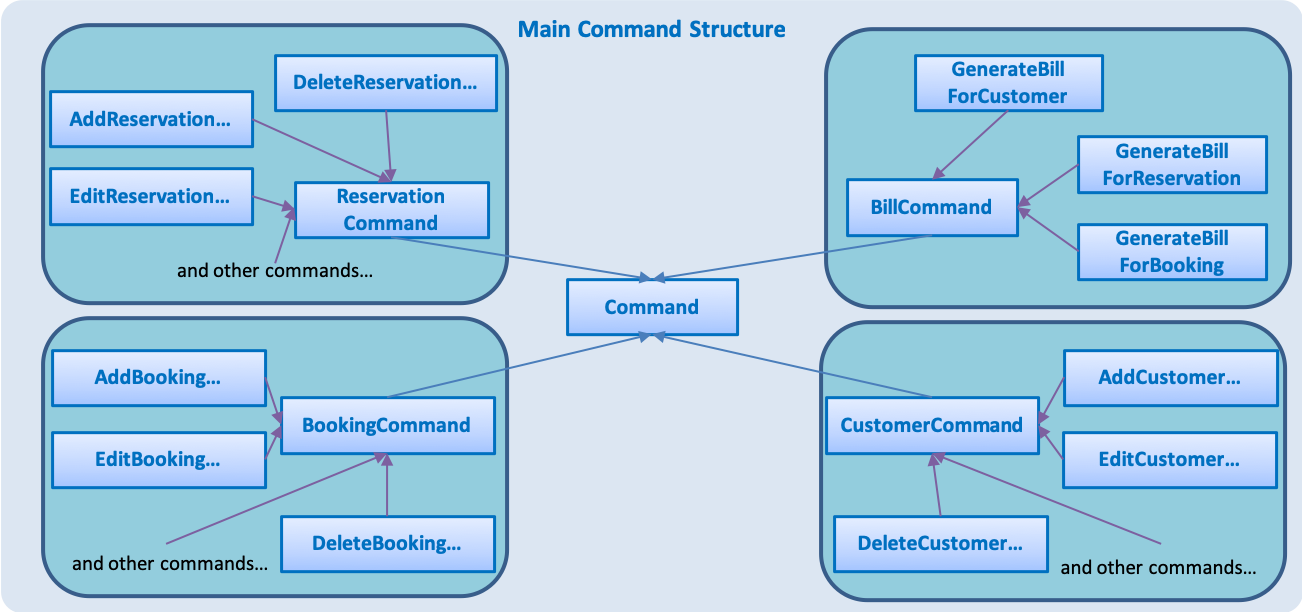
If the new model needs to be stored, then you also need to define JSON adapted classes
to represent these models. The JsonSerializableHotelManagementSystem
has a list of
JsonAdaptedCustomer
and a list of JsonAdaptedBooking
and these two lists are saved using
seedu.hms.commons.util.JsonUtil
. For each model class, you need to define a JSON-adapted
class with a toModelType
function so that the system can read the data back from the file.
The modifications in the UI for a new model depend heavily on the functionality it provides. For booking and reservations, a panel was added to display all the bookings/reservations which can be filtered based on different parameters. You can see the addition, updating and deletion of bookings in real-time in the UI as has been explained before.
Design Considerations
Aspect: How should the API be structured
-
Alternative 1 (current choice): Every model has a separate interface which implements the
Model
interface-
Pros: Every model has access to the GUI and User Preferences.
-
Cons: Some models have unnecessary methods.
-
-
Alternative 2: All the model APIs will be separate and there will be no Model Interface.
-
Pros: Better separation and less scope for errors.
-
Cons: Lot of refactoring required.
-
Making a model customizable
Current Implementation
In this section a model is considered to be customizable if an instance of it can be:
-
Added
-
Edited
-
Deleted
-
Listed (or Displayed)
You can relate this with the infamous CRUD cycle if you are familiar with it. |
Every model/manager has access to the HotelManagementSystem
inside which resides all the
lists (BookingList
, UniqueCustomerList
, etc.). These lists have methods to add an item,
set or replace an item at a particular index and delete an item. Therefore, when you are
implementing a model and its list wrapper (Eg. ReservationList
), you must include these functions.
You must also define equality between instances of a model created so that it is easy to find
an instance and replace it. Include an instance of your list inside the HotelManagementSystem
and add a listener to it to automatically update the UI every time the function indicateModified
is called.
Based on the action, you might want to update the shown list using the updateFiltered#{Model Name}List
method.
A sequence diagram is given below to make the following more clear.
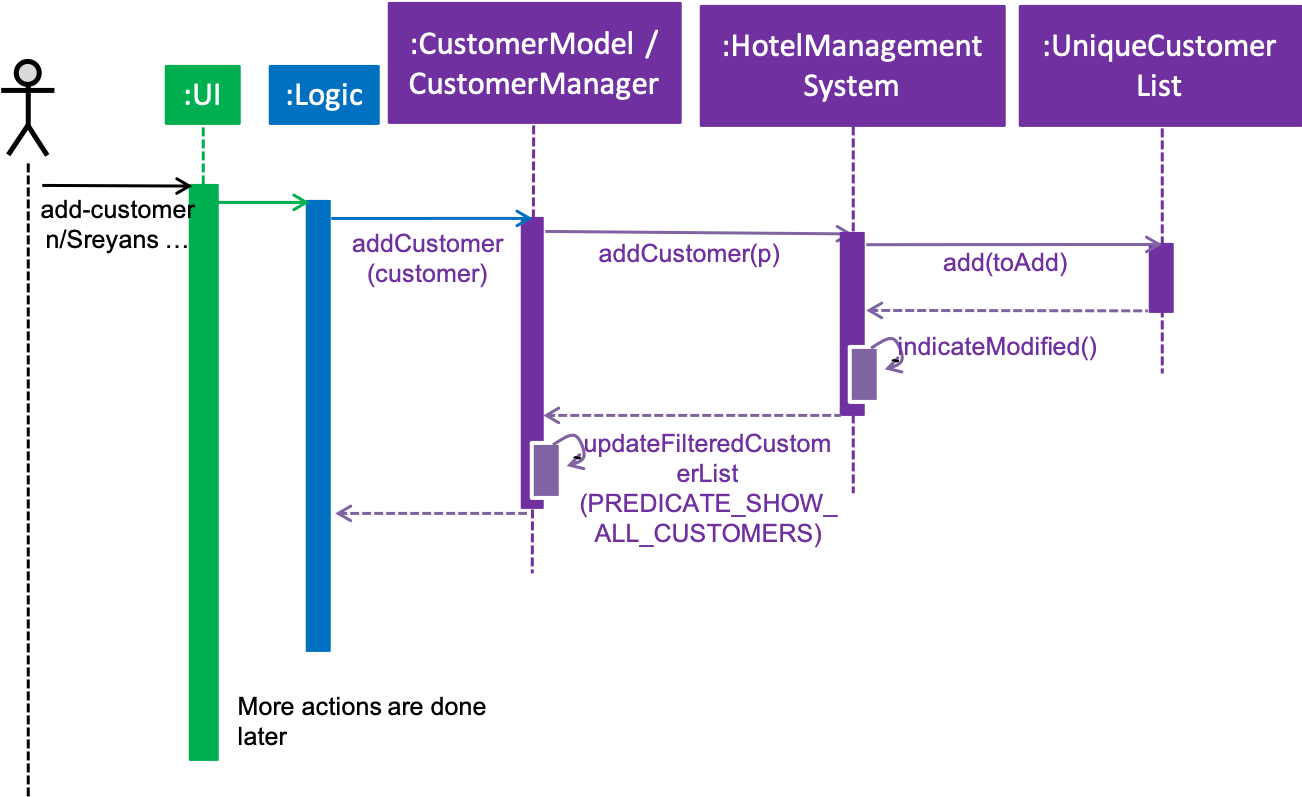
add-customer
Design Considerations
Aspect: Editing a model object
-
Alternative 1: Allowing objects to be edited
-
Pros: We avoid recreating new instances and will have better performance.
-
Cons: It is very hard to update the UI every time an object is added or updated.
-
-
Alternative 2 (current choice): Replacing objects with a new object with edited parameters
-
Pros: Lot of instances are created and we need to make sure the old object doesn’t reappear again.
-
Cons: It is easy to update the UI using a listener for each list.
-
Achievements and Learning
Given below are the my takeaways from this project and my achievements in this project. |
-
Worked with a team to implement complementing components smoothly
-
Learned how to evaluate user requirements using user stories
-
I was able to lead the module in terms of code contribution. image::reposense.png